
パンチングマシンについて
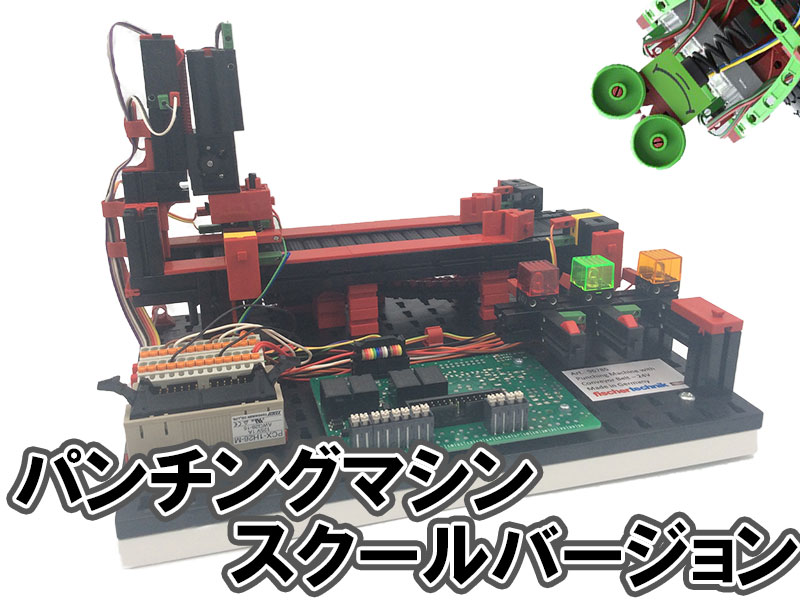
今回は最終的にfischertechnik・educationのパンチングマシンを動作させることをゴールとします!
パンチングマシンはフィッシャーテクニックの数あるシリーズの中の、『トレーニングシリーズ』というプログラミングを学ぶ方向けの製品の一つです。
パンチングマシンスクールバージョンの詳しい動画はYoutubeで公開中です!
こだわりや特徴、パンチングマシン(スクールバージョンでない)との違いなども詳しく載っています!
スイッチボード
今回はRevolutionPI(以下レボパイ)を使ってパンチングマシンを動かしますが、
いきなりパンチングマシンを動作させるのではなく、スイッチ×4 LED×4のスイッチボードを使用して入出力を割り当てるプログラムを作成してからパンチングマシンに挑みます!
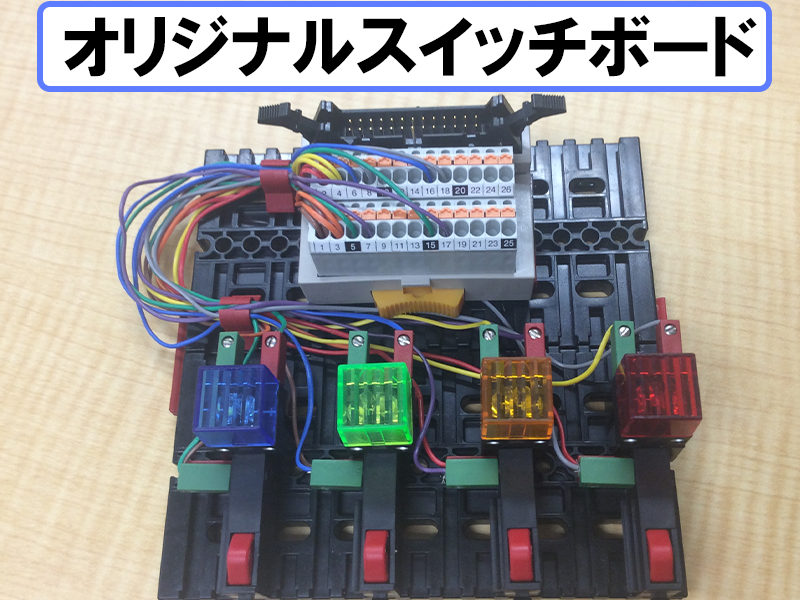
スイッチボードの詳しい内容もYoutubeで公開中です!
配線についてなども詳しく動画内で説明があるのでぜひ!
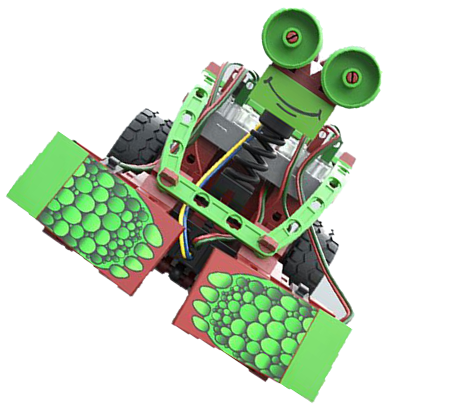
スイッチボードを実際に使用し動画はTwitterにも載せています!
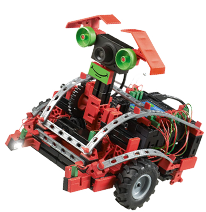
C言語で実際にプログラミング
ここからは実際にC言語でプログラミングを行っていきます!
初めてレボパイを触れる方や何をやっているのかわからない方は以前投稿した『RevoluitonPiでLチカ』のシリーズを見ていただけると理解しやすくなるかもしれません。
レボパイに電源を入れます
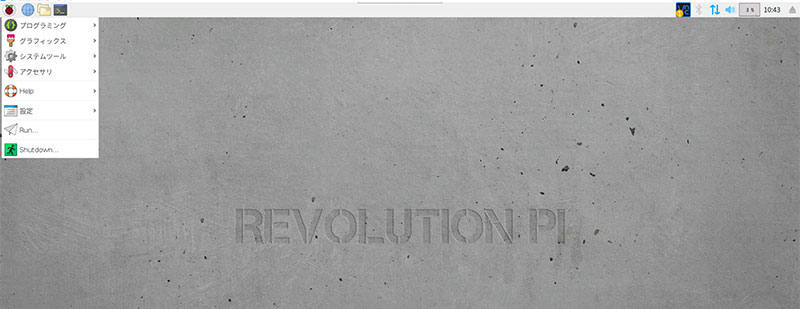
フォルダを開き、demoフォルダの中の『piTest.c』を開きます
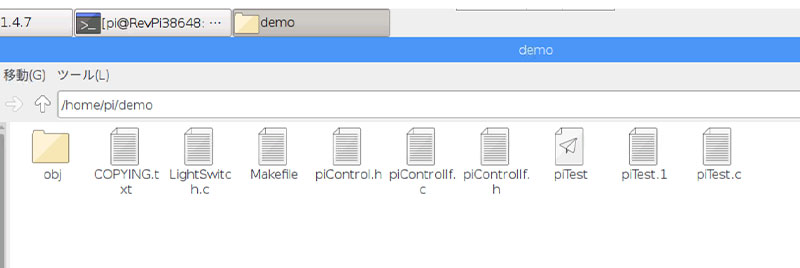
現在のプログラムは『入力×1』と『出力×1』のプログラムです。
このプログラムを『入力×4』・『出力×4』のプログラムへと変更していきます。
まずは入力・出力がそれぞれ1つのコードを確認!
- // === 1 ====
- int i = 0;
- int iLastInputValue = 0;
- char *pchInput = NULL;
- char *pchOutput = NULL;
- // structures containing variable information: Name, Offset, Bit, Length
- SPIVariable spiVariableIn = {"", 0, 0, 0};
- SPIVariable spiVariableOut = {"", 0, 0, 0};
- // structures containing variable value: Offset, Bit, Value
- SPIValue sValueIn = {0, 0, 0};
- SPIValue sValueOut = {0, 0, 0};
このコードを基にして 2~4まで合計3つ追加します。
- // === 2 ===
- int i2 = 0;
- int iLastInputValue2 = 0;
- char *pchInput2 = NULL;
- char *pchOutput2 = NULL;
- // structures containing variable information: Name, Offset, Bit, Length
- SPIVariable spiVariableIn2 = {"", 0, 0, 0};
- SPIVariable spiVariableOut2 = {"", 0, 0, 0};
- // structures containing variable value: Offset, Bit, Value
- SPIValue sValueIn2 = {0, 0, 0};
- SPIValue sValueOut2 = {0, 0, 0};
- //=== 3 ===
- int i3 = 0;
- int iLastInputValue3 = 0;
- char *pchInput3 = NULL;
- char *pchOutput3 = NULL;
- // structures containing variable information: Name, Offset, Bit, Length
- SPIVariable spiVariableIn3 = {"", 0, 0, 0};
- SPIVariable spiVariableOut3 = {"", 0, 0, 0};
- // structures containing variable value: Offset, Bit, Value
- SPIValue sValueIn3 = {0, 0, 0};
- SPIValue sValueOut3 = {0, 0, 0};
- // === 4 ====
- int i4 = 0;
- int iLastInputValue4 = 0;
- char *pchInput4 = NULL;
- char *pchOutput4 = NULL;
- // structures containing variable information: Name, Offset, Bit, Length
- SPIVariable spiVariableIn4 = {"", 0, 0, 0};
- SPIVariable spiVariableOut4 = {"", 0, 0, 0};
- // structures containing variable value: Offset, Bit, Value
- SPIValue sValueIn4 = {0, 0, 0};
- SPIValue sValueOut4 = {0, 0, 0};
これから作成する関数のプロトタイプ宣言
- int IO_1(void);
- int IO_2(void);
- int IO_3(void);
- int IO_4(void);
そして、入力のチェックと出力を行うプログラムを記述した関数
これを基にしてまた2~4を作成します。
- // ----------- 1 --------------
- int IO_1(void) {
- i = piControlGetBitValue(&sValueIn);// PiBridge - read input pin
- if(0 != i) // handle error
- {
- fprintf(stderr, "Error: piControlGetBitValue() returned %d\n", i);
- return -1;
- }
- if(iLastInputValue != sValueIn.i8uValue) // if button state changed
- { // show the change
- printf("%-32s : %d \n", pchInput, sValueIn.i8uValue);
- if(0 == sValueIn.i8uValue) // if button is released
- {
- if(1 == iLastInputValue) // and was pressed before
- { // switch light
- sValueOut.i8uValue = ~sValueOut.i8uValue;
- // PiBridge - set output pin
- i = piControlSetBitValue(&sValueOut);
- printf("%-32s : %s \n", pchOutput, sValueOut.i8uValue ? "On" : "Off");
- if(0 != i)
- {
- fprintf(stderr, "Error: piControlSetBitValue() returned %d\n", i);
- return -1;
- }
- }
- }
- }
- iLastInputValue = sValueIn.i8uValue; // remember last input value
- }
- // ----------- 2 --------------
- int IO_2(void) {
- i2 = piControlGetBitValue(&sValueIn2); // PiBridge - read input pin
- if(0 != i2) // handle error
- {
- fprintf(stderr, "Error: piControlGetBitValue() returned %d\n", i2);
- return -1;
- }
- if(iLastInputValue2 != sValueIn2.i8uValue) // if button state changed
- { // show the change
- printf("%-32s : %d \n", pchInput2, sValueIn2.i8uValue);
- if(0 == sValueIn2.i8uValue) // if button is released
- {
- if(1 == iLastInputValue2) // and was pressed before
- { // switch light
- sValueOut2.i8uValue = ~sValueOut2.i8uValue;
- // PiBridge - set output pin
- i2 = piControlSetBitValue(&sValueOut2);
- printf("%-32s : %s \n", pchOutput2, sValueOut2.i8uValue ? "On" : "Off");
- if(0 != i)
- {
- fprintf(stderr, "Error: piControlSetBitValue() returned %d\n", i2);
- return -1;
- }
- }
- }
- }
- iLastInputValue2 = sValueIn2.i8uValue; // remember last input value
- }
- // ----------- 3 --------------
- int IO_3(void) {
- i3 = piControlGetBitValue(&sValueIn3); // PiBridge - read input pin
- if(0 != i3) // handle error
- {
- fprintf(stderr, "Error: piControlGetBitValue() returned %d\n", i3);
- return -1;
- }
- if(iLastInputValue3 != sValueIn3.i8uValue) // if button state changed
- { // show the change
- printf("%-32s : %d \n", pchInput3, sValueIn3.i8uValue);
- if(0 == sValueIn3.i8uValue) // if button is released
- {
- if(1 == iLastInputValue3) // and was pressed before
- { // switch light
- sValueOut3.i8uValue = ~sValueOut3.i8uValue;
- // PiBridge - set output pin
- i3 = piControlSetBitValue(&sValueOut3);
- printf("%-32s : %s \n", pchOutput3, sValueOut3.i8uValue ? "On" : "Off");
- if(0 != i3)
- {
- fprintf(stderr, "Error: piControlSetBitValue() returned %d\n", i3);
- return -1;
- }
- }
- }
- }
- iLastInputValue3 = sValueIn3.i8uValue; // remember last input value
- }
- // ----------- 4--------------
- int IO_4(void) {
- i4 = piControlGetBitValue(&sValueIn4); // PiBridge - read input pin
- if(0 != i4) // handle error
- {
- fprintf(stderr, "Error: piControlGetBitValue() returned %d\n", i4);
- return -1;
- }
- if(iLastInputValue4 != sValueIn4.i8uValue) // if button state changed
- { // show the change
- printf("%-32s : %d \n", pchInput4, sValueIn4.i8uValue);
- if(0 == sValueIn4.i8uValue) // if button is released
- {
- if(1 == iLastInputValue4) // and was pressed before
- { // switch light
- sValueOut4.i8uValue = ~sValueOut4.i8uValue;
- // PiBridge - set output pin
- i4 = piControlSetBitValue(&sValueOut4);
- printf("%-32s : %s \n", pchOutput4, sValueOut4.i8uValue ? "On" : "Off");
- if(0 != i4)
- {
- fprintf(stderr, "Error: piControlSetBitValue() returned %d\n", i4);
- return -1;
- }
- }
- }
- }
- iLastInputValue4 = sValueIn4.i8uValue; // remember last input value
- }
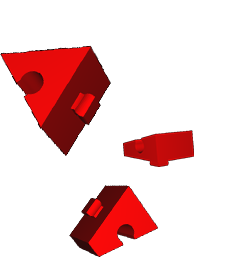
以下はmainプログラムです。
- int main(int argc, char ** argv)
- {
- pchInput = argv[1]; // PiCtory input pin for Switch
- pchOutput = argv[2]; // PiCtory output pin for Light
- pchInput2 = argv[3]; // PiCtory input pin for Switch
- pchOutput2 = argv[4]; // PiCtory output pin for Light
- pchInput3 = argv[5]; // PiCtory input pin for Switch
- pchOutput3 = argv[6]; // PiCtory output pin for Light
- pchInput4 = argv[7]; // PiCtory input pin for Switch
- pchOutput4 = argv[8]; // PiCtory output pin for Light
- strncpy(spiVariableIn.strVarName, pchInput, sizeof(spiVariableIn.strVarName));
- strncpy(spiVariableOut.strVarName, pchOutput, sizeof(spiVariableOut.strVarName));
- strncpy(spiVariableIn2.strVarName, pchInput2, sizeof(spiVariableIn2.strVarName));
- strncpy(spiVariableOut2.strVarName, pchOutput2, sizeof(spiVariableOut2.strVarName));
- strncpy(spiVariableIn3.strVarName, pchInput3, sizeof(spiVariableIn3.strVarName));
- strncpy(spiVariableOut3.strVarName, pchOutput3, sizeof(spiVariableOut3.strVarName));
- strncpy(spiVariableIn4.strVarName, pchInput4, sizeof(spiVariableIn4.strVarName));
- strncpy(spiVariableOut4.strVarName, pchOutput4, sizeof(spiVariableOut4.strVarName));
- i = piControlGetVariableInfo(&spiVariableIn); // PiBridge - get variable info
- if(0 != i) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i, spiVariableIn.strVarName);
- return -1;
- }
- i2 = piControlGetVariableInfo(&spiVariableIn2); // PiBridge - get variable info
- if(0 != i2) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i2, spiVariableIn2.strVarName);
- return -1;
- }
- i3 = piControlGetVariableInfo(&spiVariableIn3); // PiBridge - get variable info
- if(0 != i3) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i3, spiVariableIn3.strVarName);
- return -1;
- }
- i4 = piControlGetVariableInfo(&spiVariableIn4); // PiBridge - get variable info
- if(0 != i4) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i4, spiVariableIn4.strVarName);
- return -1;
- }
- // =============================
- i = piControlGetVariableInfo(&spiVariableOut); // PiBridge - get variable info
- if(0 != i) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i, spiVariableOut.strVarName);
- return -1;
- }
- i2 = piControlGetVariableInfo(&spiVariableOut2); // PiBridge - get variable info
- if(0 != i2) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i2, spiVariableOut2.strVarName);
- return -1;
- }
- i3 = piControlGetVariableInfo(&spiVariableOut3); // PiBridge - get variable info
- if(0 != i3) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i3, spiVariableOut3.strVarName);
- return -1;
- }
- i4 = piControlGetVariableInfo(&spiVariableOut4); // PiBridge - get variable info
- if(0 != i4) // handle error
- {
- fprintf(stderr, "Error: piControlGetVariableInfo() returned %d for variable '%s' \n",
- i4, spiVariableOut4.strVarName);
- return -1;
- }
- // =============================
- sValueIn.i16uAddress = spiVariableIn.i16uAddress;
- sValueIn.i8uBit = spiVariableIn.i8uBit;
- sValueIn.i8uValue = 0;
- sValueOut.i16uAddress = spiVariableOut.i16uAddress;
- sValueOut.i8uBit = spiVariableOut.i8uBit;
- sValueOut.i8uValue = 0;
- // --------------- 2
- sValueIn2.i16uAddress = spiVariableIn2.i16uAddress;
- sValueIn2.i8uBit = spiVariableIn2.i8uBit;
- sValueIn2.i8uValue = 0;
- sValueOut2.i16uAddress = spiVariableOut2.i16uAddress;
- sValueOut2.i8uBit = spiVariableOut2.i8uBit;
- sValueOut2.i8uValue = 0;
- // ----------------- 3
- sValueIn3.i16uAddress = spiVariableIn3.i16uAddress;
- sValueIn3.i8uBit = spiVariableIn3.i8uBit;
- sValueIn3.i8uValue = 0;
- sValueOut3.i16uAddress = spiVariableOut3.i16uAddress;
- sValueOut3.i8uBit = spiVariableOut3.i8uBit;
- sValueOut3.i8uValue = 0;
- // ------------------- 4
- sValueIn4.i16uAddress = spiVariableIn4.i16uAddress;
- sValueIn4.i8uBit = spiVariableIn4.i8uBit;
- sValueIn4.i8uValue = 0;
- sValueOut4.i16uAddress = spiVariableOut4.i16uAddress;
- sValueOut4.i8uBit = spiVariableOut4.i8uBit;
- sValueOut4.i8uValue = 0;
- // -----------------
- printf("%s is running waiting for switch '%s' \n", argv[0], pchInput);
- printf("%s is running waiting for switch '%s' \n", argv[1], pchInput2);
- printf("%s is running waiting for switch '%s' \n", argv[2], pchInput3);
- printf("%s is running waiting for switch '%s' \n", argv[3], pchInput4);
- while(1)
- {
- IO_1();
- IO_2();
- IO_3();
- IO_4();
- }
- return 0;
- }
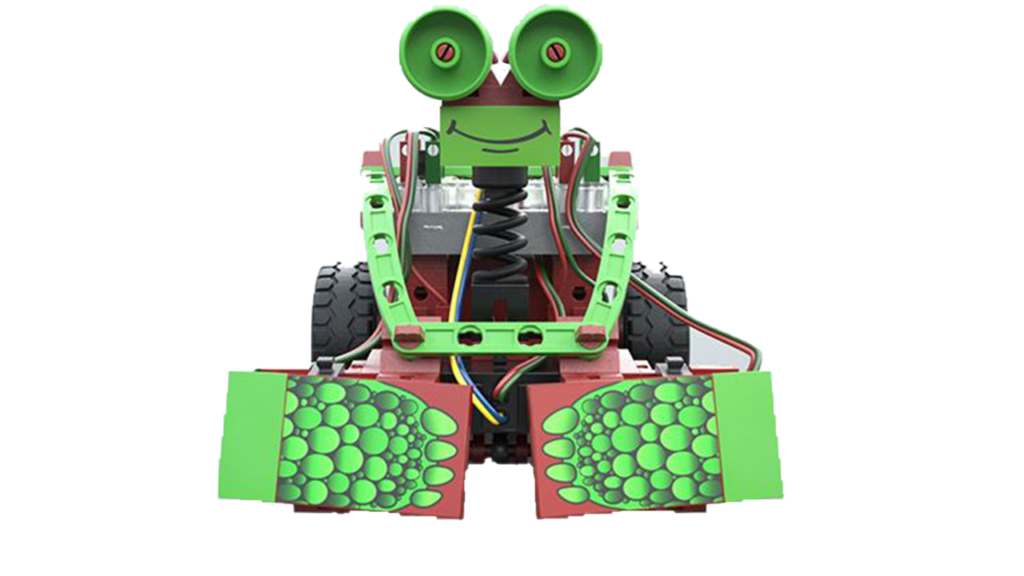
プログラムが完成したのでレボパイとスイッチボードをリボンケーブルで接続します
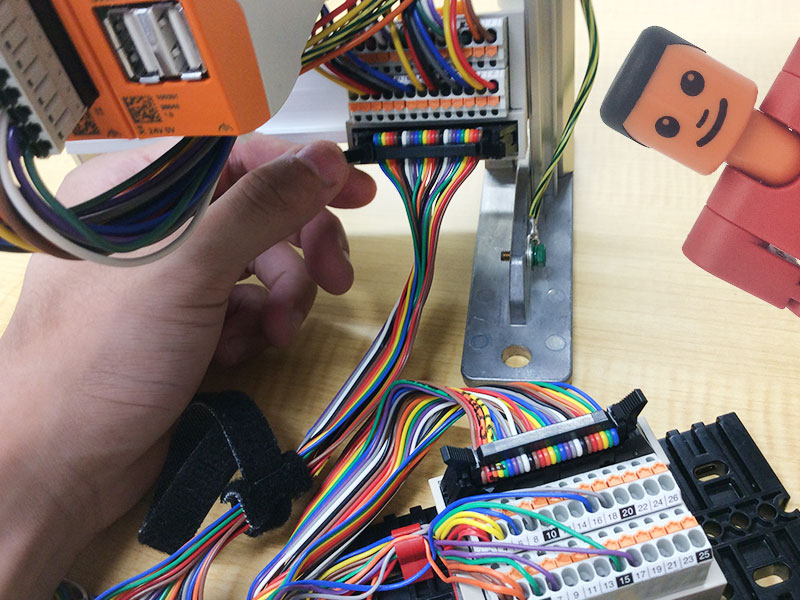
ターミナルで実行
ターミナルをデスクトップ画面から立ち上げます
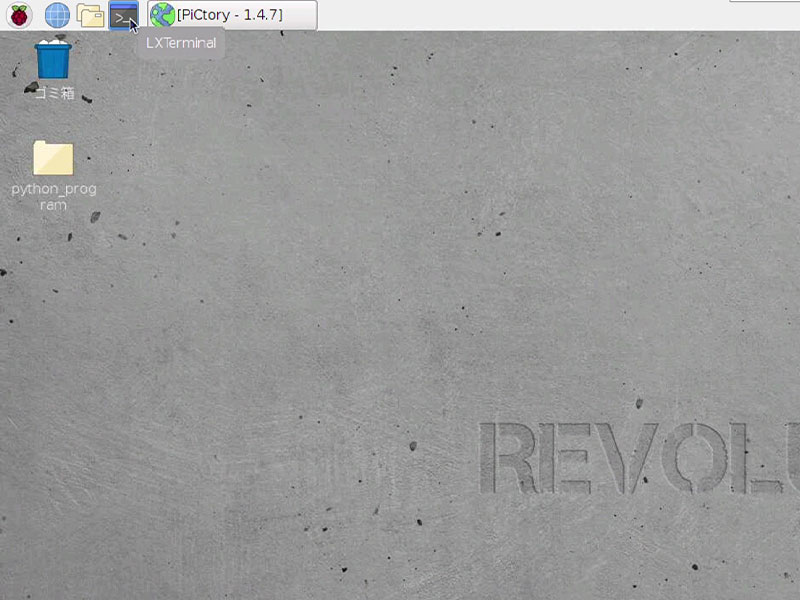

cd demo

makeコマンド でコンパイルします
make

./piTest I_1 O_1 I_2 O_2 I_3 O_3 I_4 O_4

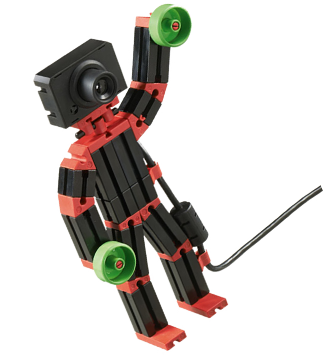
スイッチボードの出力は『LED』ですが、DCモーターへと簡単に変更できるので確認も容易でした!
ご覧いただきありがとうございました!
